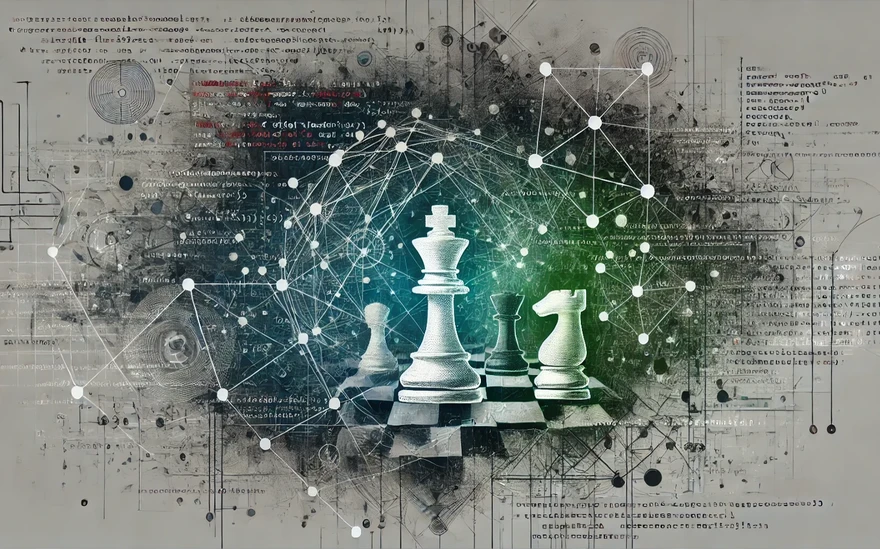
Chess Web Programming: Part One: Getting Started
Getting Started with Chess Web Application ProgrammingIn October 2023, I began learning modern web programming and used Chessboard Magic as my first major project. What started as a way to practice my skills has grown into something much larger—Chessboard Magic now includes 34 different chess-based applications! Building it was both a rewarding challenge and a fantastic way to dive deeper into web development, and now I’m excited to share this journey with you.
This guide is the first in a series that will walk you through every step, from setting up the board layout to enabling piece movement and adding interactive game logic. Whether you're here as a chess lover or simply curious about coding a board game, I hope these steps inspire you to create something of your own.
Getting Started
In this first part, we’ll set up the foundation for our Chess Web Application using React, one of the most popular web frameworks. React allows us to create dynamic and responsive user interfaces, making it an ideal choice for building an interactive chessboard. To help us manage the chessboard visuals and functionality, we’ll leverage React-Chessboard, a powerful library for rendering chessboards in React applications. Additionally, we’ll use Chess.js, a JavaScript library that handles the game’s rules and logic, enabling us to validate moves and manage game state with ease.
By the end of this section, you’ll have a basic React application set up, complete with an interactive chessboard.
Note: This guide was written with Windows users in mind.
Software
Before we dive into coding, let’s set up the tools we’ll use for this project. We’ll work within Microsoft Visual Studio Code (VS Code), a powerful and widely used code editor, and we’ll use Node Package Manager (NPM) to manage the libraries and dependencies that bring our application to life.
1. Microsoft Visual Studio Code (VS Code)
VS Code is a free, open-source code editor developed by Microsoft. It’s popular among developers because of its extensive features, customizable extensions, and support for various programming languages. For this guide, we’ll assume you’re using VS Code as your main workspace for writing, organizing, and debugging code.
Installation:
- Go to the official VS Code website.
- Click on the Download for Windows button (or choose your operating system).
- Once downloaded, open the installer and follow the installation prompts.
- After installation, launch VS Code. You can also explore additional extensions later to enhance your coding experience with features like code formatting, debugging, and more.
2. Node.js and NPM (Node Package Manager)
Node.js is a JavaScript runtime that lets you run JavaScript outside the browser, while NPM is the default package manager for Node.js. NPM allows us to install and manage third-party libraries that we’ll use to build our application, like React, React-Chessboard, and Chess.js.
Installation:
- Go to the official Node.js website.
- Download the LTS (Long Term Support) version, which includes NPM.
- Run the downloaded installer and follow the prompts to complete the installation.
3. Using the Terminal to Check Installation
After installing Node.js and NPM, let’s verify that they’re set up correctly using the terminal in VS Code. The terminal (or command-line interface) allows you to type text commands to interact with your computer’s operating system—a tool we’ll use throughout this guide for running scripts, installing libraries, and more.
To open the terminal in VS Code:
- Open VS Code.
- Click on Terminal in the top menu, then select New Terminal.
- A terminal window will appear at the bottom of your VS Code screen.
In this terminal, type the following commands to check that Node.js and NPM are installed:
- Check Node.js version: Type
node -v
and press Enter. You should see a version number (e.g., v18.14.0), which confirms that Node.js is installed. - Check NPM version: Type
npm -v
and press Enter. This will show the NPM version, indicating that NPM is also installed.
If both commands display version numbers, you’re all set! We’ll be using VS Code and the terminal regularly, so having them set up will ensure a smooth experience as we build our Chess Web Application.
Note: If you encounter the error: npm.ps1 cannot be loaded because running scripts is disabled on this system
This happens because PowerShell has restrictions on script execution for security reasons. To resolve it, you can enable script execution with these steps:
- Open PowerShell as Administrator: Search for "PowerShell" in the Start menu, right-click on it, and select Run as Administrator.
- Set Execution Policy: In the PowerShell window, run the following command:
Set-ExecutionPolicy RemoteSigned -Scope CurrentUser
- Confirm: When prompted, type
Y
and press Enter to allow the change.
Creating the Project
Now that we have our tools set up, let’s start by creating a new React application and installing the libraries we need: react-chessboard and chess.js. React will provide the foundation for our app, react-chessboard will render the chessboard, and chess.js will handle the game logic.
Step 1: Create a New React Application
We’ll use Create React App, a popular toolchain for quickly setting up a React project with a basic file structure and configuration.
1. Open VS Code and go to the Terminal
- Click on Terminal in the top menu and select New Terminal.
- This will open the terminal at the bottom of the VS Code window, allowing you to run commands directly within VS Code.
2. Navigate to the directory where you want to create the project. For example:
cd path\to\your\projects
Note on Terminal Navigation (Windows):
- cd [folder-name]: Changes the current directory to the specified folder.
- cd ..: Moves up one level in the directory structure (back to the parent folder).
- dir: Lists the files and folders in the current directory.
- Run the following command to create a new React application:
npx create-react-app myfirstchessapp
- npx allows us to run npm packages without needing to install them globally.
- create-react-app is a tool that sets up a new React project with a standard structure and configuration files.
- myfirstchessapp is the name of your project folder. You can replace it with any name you like.
4. Open the Project Folder in VS Code:
To open the folder directly from within VS Code, you can click on File > Add Folder to Workspace... and select the myfirstchessapp folder.
5. Navigate into the project directory (if you’re not already in it):
cd myfirstchessapp
6. To verify that everything works, run the app:
npm start
This will start a development server and open the app in your default browser. You should see a basic React page with a welcome message, confirming that the project is set up correctly.
Step 2: Install React-Chessboard and Chess.js
Now that we have our React application ready, let’s install react-chessboard and chess.js.
1. In the terminal, make sure you’re in your project directory (myfirstchessapp) and run the following command:
npm install react-chessboard chess.js
- react-chessboard is a React component that makes it easy to display a chessboard in your application. It handles all the graphical representation of the board and pieces.
- chess.js is a JavaScript library that provides the rules and logic for chess. It can validate moves, track the game state, and detect checkmates or stalemates.
By installing these libraries, we’re adding them to our project’s dependencies, which will allow us to import and use them in our code.
Step 3: Check the Installed Packages
To confirm that react-chessboard and chess.js were installed correctly, you can check the package.json
file in the root of your project directory.
1. Open package.json
in VS Code.
2. Look for the dependencies section, which should include entries for react-chessboard and chess.js. It should look something like this:
"dependencies": {
"react": "^18.0.0",
"react-dom": "^18.0.0",
"react-scripts": "5.0.0",
"react-chessboard": "^1.4.0",
"chess.js": "^1.0.0"
}
With these libraries installed, you’re ready to start building the chessboard in the next steps! We’ll use react-chessboard to create an interactive board, and chess.js to handle the rules and logic of the game.
Developing the Application
With our project and dependencies set up, let’s start building the core functionality of our chess application. In this section, we’ll edit the App.js
file to display a chessboard using react-chessboard and validate moves using chess.js. This will allow us to ensure that only legal moves are accepted on the board and handle any errors for invalid moves gracefully.
Step 1: Set Up App.js
1. In VS Code, open the App.js
file located in the src
folder of your project.
2. Replace the contents of App.js
with the following code to import react-chessboard and chess.js and set up a basic chessboard component with move validation and a fixed width.
import React, { useState } from 'react'; // Import React and useState to manage state
import { Chessboard } from 'react-chessboard'; // Import Chessboard component from react-chessboard
import { Chess } from 'chess.js'; // Import Chess logic from chess.js
// Main App component
const App = () => {
// Initialize the game state using useState with a new Chess instance
const [game, setGame] = useState(new Chess());
// Function to handle piece movement on the chessboard
const onDrop = (sourceSquare, targetSquare) => {
// Create a copy of the current game state using FEN notation
const gameCopy = new Chess(game.fen());
try {
// Attempt to make the move on the game copy
const move = gameCopy.move({
from: sourceSquare, // Starting square of the move
to: targetSquare, // Target square of the move
promotion: 'q' // Always promote to a queen for simplicity
});
// If the move is invalid, move will be null, so we return false to ignore the move
if (move === null) {
return false;
}
// If the move is valid, update the game state with the new position
setGame(gameCopy);
return true; // Return true to indicate a valid move
} catch (error) {
// Catch and log any errors that occur during the move attempt
console.error(error.message);
return false; // Return false to ignore the invalid move
}
};
return (
<div>
<h1>Chess Game</h1>
<Chessboard
position={game.fen()} // Set the chessboard position to the current game state
onPieceDrop={onDrop} // Trigger the onDrop function when a piece is moved
boardWidth={500} // Set the width of the chessboard to 500px
/>
</div>
);
};
export default App; // Export the App component as the default export
Code Explanation
The code comments explain each line, making it clear what each part of the code does, however,
- Imports: We import
React
anduseState
from React,Chessboard
from react-chessboard, andChess
from chess.js. - App Component:
useState(new Chess())
initializes a new chess game in thegame
state.
- onDrop Function: This function validates and handles piece movements.
- A copy of the game state,
gameCopy
, is created to test moves. move
is used to try the move; ifmove
isnull
, it’s invalid.- Errors are caught with
try-catch
, andfalse
is returned for invalid moves.
- A copy of the game state,
- Chessboard Component:
position
sets the board position usinggame.fen()
.onPieceDrop
callsonDrop
whenever a piece is moved.boardWidth={500}
sets the chessboard size to 500 pixels.
- Exporting the App Component:
App
is exported as the default so it can be used in other parts of the project.
In the terminal, start the development server if it’s not already running:
npm start
To stop the server after running npm start
, use:
Ctrl + C
in the terminal where the server is running. When prompted to terminate the batch job, confirm by typing Y
if necessary. This command will gracefully shut down the server.
Step 2: Run the Application
Open your browser to view the application. You should see a chessboard with a fixed width of 500px
and be able to move pieces. Each move will be validated by chess.js, allowing only legal moves on the board.
With this setup, you now have an interactive chessboard that validates moves. When a piece is moved, chess.js checks whether the move is legal. If it is, the move is applied; if not, the piece remains in its original position, and any errors are handled without breaking the application.
This provides a solid foundation for further enhancements to your chess application.
Summary
In this guide, we walked through the initial steps of creating a Chess Web Application using React, react-chessboard, and chess.js. Starting from setting up the development environment, we covered how to create a new React application and install the necessary libraries. We then built a functional chessboard by integrating react-chessboard for the board display and chess.js for move validation. Along the way, we implemented error handling to ensure invalid moves are gracefully managed, providing a solid foundation for building a chess application.
This is just the start. In subsequent blog posts, I’ll dive deeper by covering topics like integrating Stockfish (a powerful chess engine), exploring more features of react-chessboard and chess.js, and adding new functionality. Through these steps, I hope to encourage and inspire you to explore your own chess projects and push the boundaries of what you can build.
Learn More
- React: A popular JavaScript library for building user interfaces, especially single-page applications. React.js Documentation
- react-chessboard: A React component for rendering chessboards, offering a range of customization options for building interactive chess applications. react-chessboard GitHub Repository
- chess.js: A JavaScript library that provides chess move validation, game state management, and more. chess.js Documentation
More blog posts by HollowLeaf
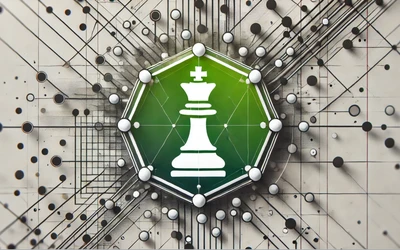
Chess Web Programming: Part Eight: Chess.com API
Working with the Chess.com API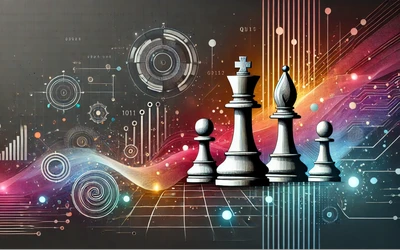
Chess Web Programming: Part Seven: Lichess API
Working with the Lichess API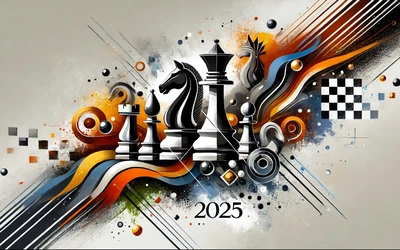
Chessboard Magic: January 2025 Update
Hello Chess Lovers, As we inch closer to 2025, I’m more excited than ever about the future of Chessb…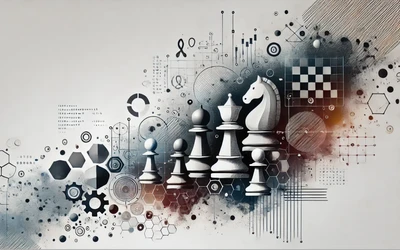