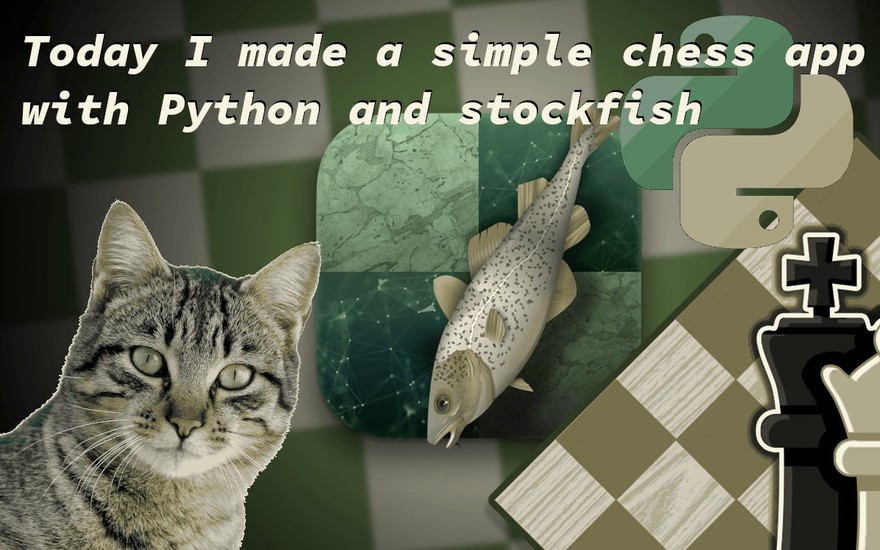
gimp / feline / stockfish / logo python / chesslogo
Creating a very simple chess App with Python and Stockfish
Using python , stockfish to create an App is fun. As a beginner in python I explain in this blog how I did it.Making an App ?
What to do to enhance my game, I pondered? Yesterday, I delved into the labyrinth of openings. Yet, to my dismay, it seemed to unleash a torrent of errors upon the board. How could this be? Every effort to refine my skills only seemed to plunge me deeper into the abyss. Frustrated and bewildered, I sought solace in casual games.
In the last years, I've tried every thing under the sun to become a better player, apart from eating a lot of chilli peppers to become a grandmaster. But as it turns out, it seems that nothing really works. My rating stays more or less the same.
In a way, I think rating doesn't change much after a certain (chess) age, but I might be wrong. What do you think? is it because the algorithm in my brain is fixed ? And when I learn new things, it simply doesn't have any long-term impact.
Yes, I know that improving is impossible when playing bullet or blitz, so should I play longer games, or is there another way to improve apart from?
Well, okay, I already talked about the CBOCM (which is the idea of learning moves without move order in a single position). But maybe Python, that metaphorical trusty snake (programming language), can come to the rescue. And so I got the idea of creating my own app with the goal to understand the thinking process better....
As a beginner in python i started to install Pycharm again because some how my cat had deleted it (how did she do it?), maybe the reason was because cats don't like cucumbers and snakes ?
In my Ubuntu box I can use the Pycharm community download version. But there is also a windows version Pycharm download windows I guess. using flatpak as package manager makes installing the snake language pretty easy. So with that IDE in place i started to 'program' my app....
Python and Chess.
As it turned out python likes chess and there is already a library called python-chess. that is very easy to install. In my Ubuntu box terminal I just had to type:
pip install python-chess o
After that i did the dishes fed my feline friend and started to code in the Pycharm IDE.....
First steps
I am really amazed how easy it is to write an chess app in python ! But what would the app do was the question.
A idea:
I a way i want to write an app that reads chess positions and searches for patterns and associates those patterns with a name. like for instance 2 rooks on a row get the association twin-towers. that way it's easier to remember certain positions
But as it turned out , this is way to complex for a python beginner , so first steps first.
just import everything there is in the chess library (I know this is not the pythonic way)
from chess import *
next step is easy too :
board = Board()
Now the Board object is instantiated, and I can print out the board with `print(board)`. As it turned out, the board isn't empty. And even in the manual, there was no explanation of how to enter a position.
Eventually, I found the amazing:
board.set_piece_map(piece_map)
All i had to do was , to chase my cat away in front of my monitor and define a piece_map which is a map object in python:
piece_map = {
A1: Piece(ROOK, WHITE), E1: Piece(KING, WHITE),
E8: Piece(KING, BLACK),H8: Piece(ROOK, BLACK),
}
Now I executed set_piece_map and got the desired position. After some tinkering and RTFM I created with
the help of using list comprehension:
piece_map = {
'abcdefgh'.index(s[1]) + '12345678'.index(s[2]) * 8: Piece(PIECE_SYMBOLS.index(s[0].lower()), s[0].isupper()) for s
in ['Qe2','ra1']}
The list ['Qe2','ra1'] can be changed to any desired position!
Connecting a Fish to a snake in a penguin ! (stockfish python linux)
Well the board cannot do much when it come to playing moves. I am quite lucky because i live in the original Stockfish city in the Netherlands(home of the guy that invented REBEL chess long long age) but connecting that fish to the snake code turned out to be quite difficult. First I downloaded stockfish
but for some strange reason i accidentally downloaded a older version. tried to Make the fish but got a lot of errors. Luckily i discovered the mistake and after downloading version 16.1 it worked.
However stockfish is based on UCI , which is a chess protocol and i assumed i had to download another library just to interface with python. it turned out to be a wild goose chase because there is also a module that interfaces with the chess board in python directly !!!
import chess.engine
Amazing don't you think !, now i just have to tell python and chess how to use the module...
engine = chess.engine.SimpleEngine.popen_uci(r"/home/ricky/bin/scid_linux/engines/stockfish-ubuntu-x86-64-avx2/stockfish/stockfish-ubuntu-x86-64-avx2")
board = Board()
info = engine.analyse(board, chess.engine.Limit(time=0.1),multipv=10)
info contains now the multipv info , that is the info of the 10 best moves for a given position...
print("Score:", info[0]['pv'])
Now I can print out the 10 best moves !
however printing out the chessboard is ugly and i want an interface , like a window , and an avatar of my cat if possible. so I need another library called tkinter
With tkinter it's possible to put a label on a window and give the label an chessimage that represents the chessboard. easy ...
import tkinter as tk
root =tk.TK()
label = tk.Label(root)
label.pack() # pack the label into the root
and with...
import chess.svg
svg_board= chess.svg.board(board,size=640,coordinates=False)
It's possible to make an svg board (that is a graphical representation) of the current chess position.
However it's not possible to use a svg directly in tkinter so some conversion has to take place.
render_svg = cairosvg.svg2png(bytestring=svg_board)
from PIL import Image, ImageTk
import cairosvg
from io import BytesIO
image = Image.open(BytesIO(render_svg))
photo_image = ImageTk.PhotoImage(image)
The next thing is it to add a button to the window and write a def that executes code when the button is pushed. as a test i used the following code :
def random_chess_position(n_ply=12):
board = chess.Board()
engine = chess.engine.SimpleEngine.popen_uci(r"/home/ray/bin/scid_linux/engines/stockfish-ubuntu-x86-64-avx2/stockfish/stockfish-ubuntu-x86-64-avx2")
for n in range(n_ply):
result = engine.play(board, chess.engine.Limit(time=0.1))
board.push(result.move)
engine.quit()
#board.push(choice(list(board.legal_moves))) svg_board= chess.svg.board(board,size=640,coordinates=False)
render_svg = cairosvg.svg2png(bytestring=svg_board)
image = Image.open(BytesIO(render_svg))
photo_image = ImageTk.PhotoImage(image)
label.photo = photo_image
label.config(image=photo_image)
root.after(500,random_chess_position)
But there was a little problem by executing the code. and that was because python did garbage collectionon the photo image. to prevent that (i actually had to ask Chatgpt for help)
label.photo = photo_image is necessary. this is because the label.photo variable creates a reference count outside the function
After 4 hours my little app worked. it created random chess positions generated by Stockfish. Not very useful i must admit but i learned a lot in that time. In a way this is a starting point for more useful apps. like for instance a Webserver (using flask) that generates mate patterns.
Suddenly my cat jumped in front of the monitor demanding attention. i looked on the clock "already 12'o clock' time to go to bed. another day went by. I learned useful skills.
So what do you think ? is it a useful skill to know to create a chess app.
Greetings,
More blog posts by rickyVONicky
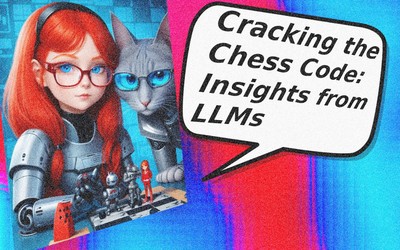
Cracking the Chess Code: Insights from LLMs
I felt trapped in a cycle of frustration, rating fluctuations , and unable to improve my game I disc…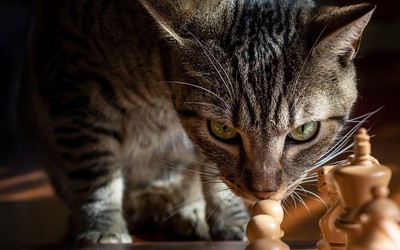
My Blundering in chess stopped , but why ?
still puzzled why for 2 weeks i blundered my way down the ladder, suddenly i stopped blundering but …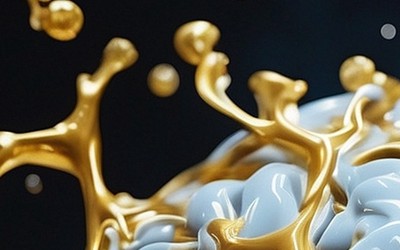