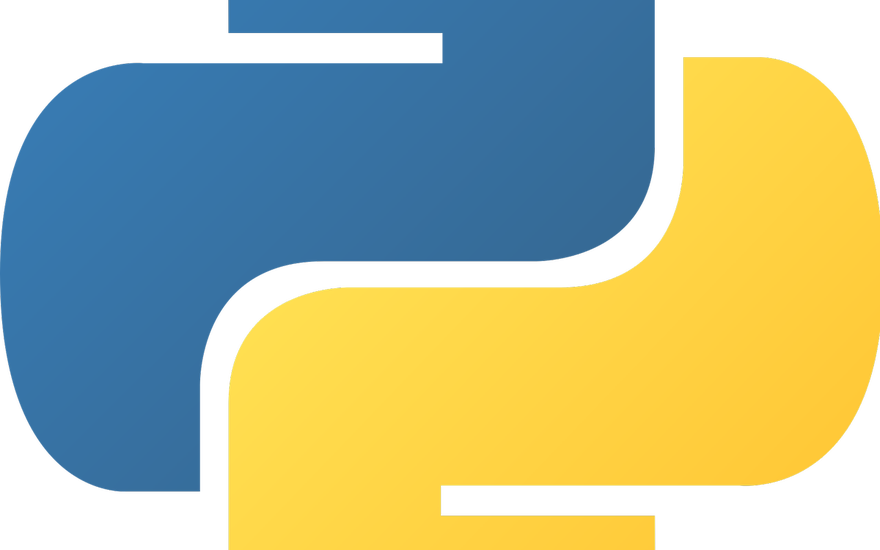
Python in five minutes
In this blog, I will be teaching python basics in a short blog, it should be helpfull if you want to get into the IT field and you niether have the time not know where to startPython is a popular programming language that is easy to learn and has a wide range of applications. Whether you are interested in web development, data analysis, machine learning, or automation, Python can be a powerful tool. In this blog, we will cover all the basics of Python programming, including variables, data types, control structures, functions, and modules.
Variables
A variable is a named storage location in memory that can hold a value. In Python, variables are created by assigning a value to a name using the equals sign (=). For example, the following code creates a variable named x and assigns it the value 5:
x = 5
Data Types
Python has several built-in data types, including integers, floats, booleans, strings, lists, tuples, and dictionaries.
Integers are whole numbers, such as 1, 2, 3, etc.
Floats are numbers with a decimal point, such as 3.14 or 2.0.
Booleans are either True or False.
Strings are sequences of characters, enclosed in quotes, such as "Hello, World!".
Lists are ordered collections of items, enclosed in square brackets, such as [1, 2, 3].
Tuples are ordered collections of items, enclosed in parentheses, such as (1, 2, 3).
Dictionaries are unordered collections of key-value pairs, enclosed in curly braces, such as {"name": "John", "age": 30}.
Control Structures
Python has several control structures that allow you to control the flow of your program. These include if statements, for loops, while loops, and try-except blocks.
If statements allow you to execute code only if a certain condition is true. For example:
if x > 0:
print("x is positive")
For loops allow you to iterate over a collection of items, such as a list. For example:
numbers = [1, 2, 3, 4, 5]
for number in numbers:
print(number)
While loops allow you to execute code repeatedly as long as a certain condition is true. For example:
x = 0
while x < 10:
print(x)
x = x + 1
Try-except blocks allow you to handle errors gracefully. For example:
try:
x = int(input("Enter a number: "))
except ValueError:
print("That's not a number!")
Functions
Functions allow you to break your code into reusable pieces that can be called from other parts of your program. Functions are created using the def keyword, followed by the name of the function and any parameters it takes. For example:
def add(x, y):
return x + y
Modules
Python has a vast library of modules that provide additional functionality beyond the built-in data types and control structures. To use a module, you need to import it using the import keyword. For example:
import math
print(math.pi)
Conclusion
Python is a versatile and powerful programming language that can be used for a wide range of applications. In this blog, we covered all the basics of Python programming, including variables, data types, control structures, functions, and modules. With these fundamentals under your belt, you will be well on your way to becoming a proficient Python programmer.
A big thank to chat gpt for helping me to make this blog
More blog posts by heckerboy
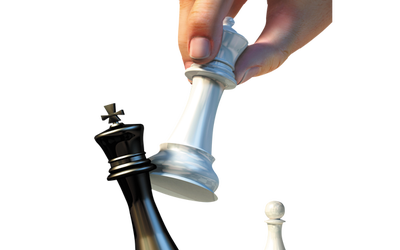
Guess the ELO ep1
I know it is like Levy's videos, but hey we are doing our best okay ? anyways in this series we will…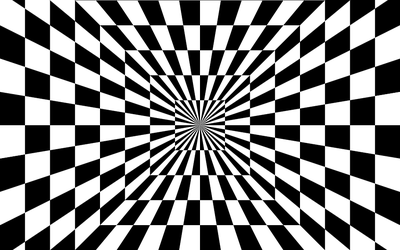
How to not hung peices in chess
Have you ever hung your queen in a middle of a game evn if you were totally focused, this blog will …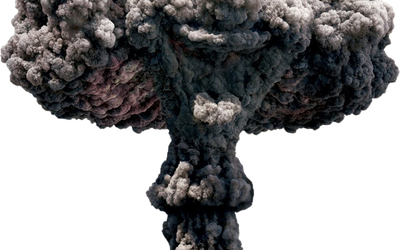
This Man Saved your Life and Mine
If it was not for him, we would probably be both dead, but who is he?